Hi, Readers.
In this post, I would like to briefly talk about how to flow/pass custom field values in Business Central. Generally, it is from the table before posting to the posted table. And of course there will be some special needs.
I keep seeing people asking similar questions in the Business Central forum, and in order to help more people, I will share some solutions in this post. (I will continue to update)
PS: Please note that the following may not be the only solution. Subscribing to other events can also be done. You can modify the code according to your needs.
- TransferFields Method
- From Item Journals (83 Item Journal Line) to Item Ledger Entries (32 Item Ledger Entry)
- From Purchase Order Subform (39 Purchase Line) to Item Ledger Entries (32 Item Ledger Entry)
- From Whse. Receipt Subform (7317 Warehouse Receipt Line) to Item Ledger Entries (32 Item Ledger Entry)
- From Sales Journal (81 Gen. Journal Line) to Customer Ledger Entries (21 Cust. Ledger Entry)
- From Purchase Journal (81 Gen. Journal Line) to Vendor Ledger Entries (25 Vendor Ledger Entry)
- From Purchase Journal (81 Gen. Journal Line) to General Ledger Entries (17 G/L Entry)
- From Sales Order (36 Sales Header) to General Ledger Entries (17 G/L Entry)
- From Purchase Order (50 Purchase header) to Warehouse Receipt (7316 Warehouse Receipt Header)
- From Warehouse Shipment (7320 Warehouse Shipment Header) to Posted Sales Shipment (110 Sales Shipment Header)
- From Bank Acc. Reconciliation Lines (274 Bank Acc. Reconciliation Line) to General Journal (81 Gen. Journal Line)
TransferFields Method
The TransferFields
method copies fields based on the field number on the fields. For each field in Record
(the destination), the contents of the field that has the same field number in FromRecord
(the source) will be copied, if such a field exists. The fields must have the same data type for the copying to succeed (text and code are convertible, other types are not). Enum fields are considered being the same data type even on different enum types. There must be room for the actual length of the contents of the field to be copied in the field to which it is to be copied. If any one of these conditions are not fulfilled, a runtime error will occur. More details: Record.TransferFields(var Record [, Boolean]) Method: Copies all matching fields in one record to another record.
For the following tables related to Sales and Purchase, we only need to add the same fields (same field number and same data type).
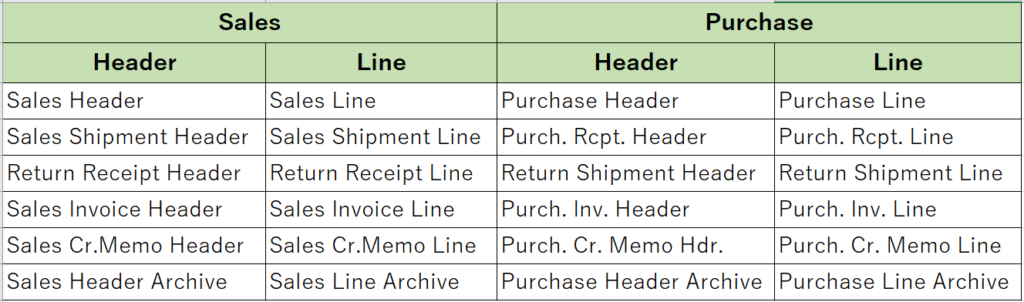
For example,
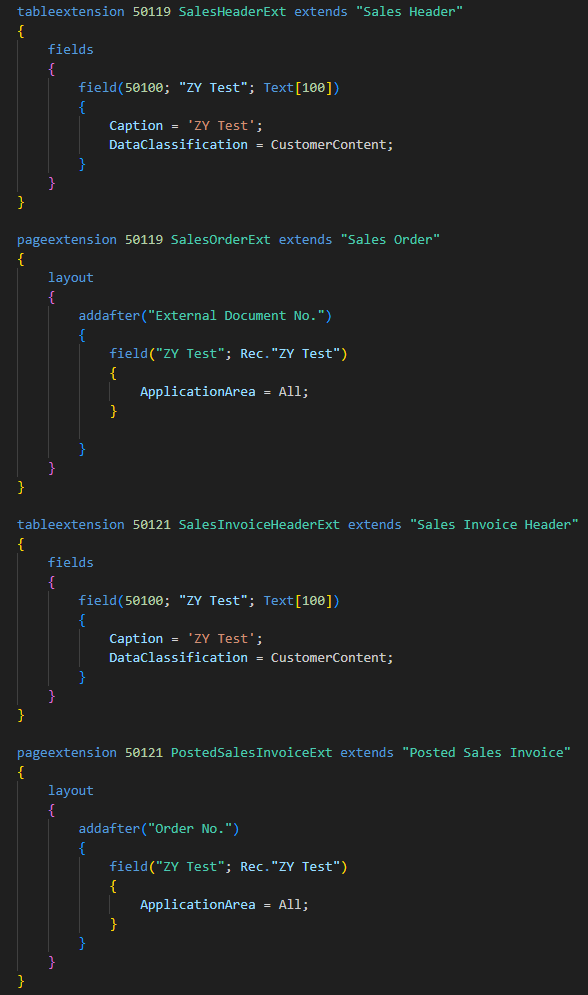
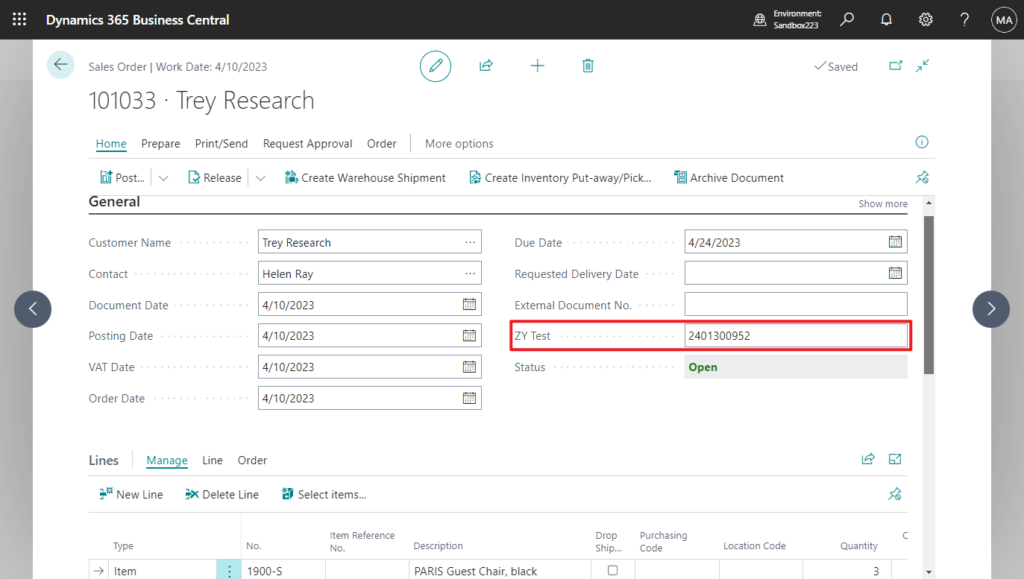
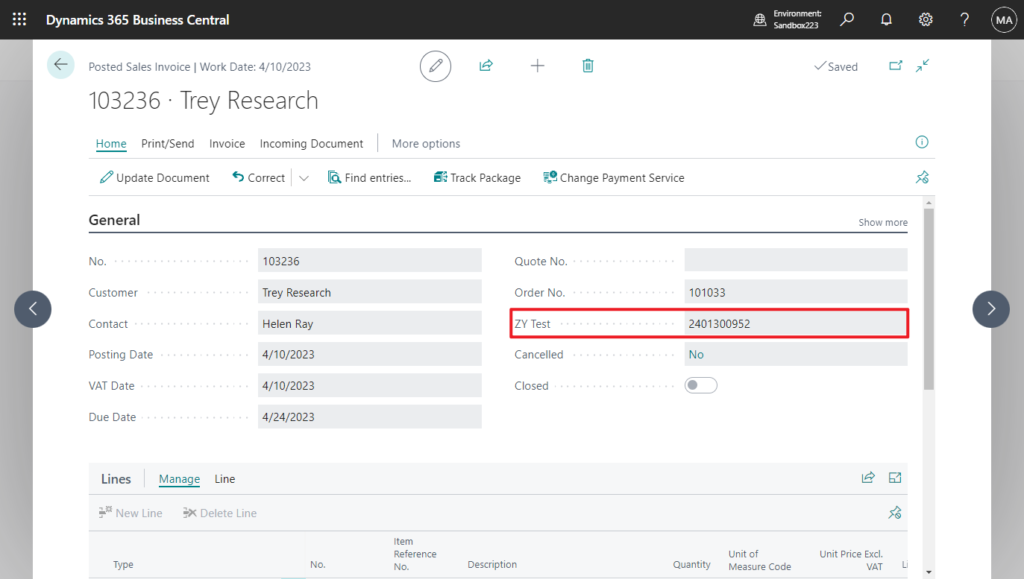
Source code:
tableextension 50119 SalesHeaderExt extends "Sales Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 SalesOrderExt extends "Sales Order"
{
layout
{
addafter("External Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50121 SalesInvoiceHeaderExt extends "Sales Invoice Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 PostedSalesInvoiceExt extends "Posted Sales Invoice"
{
layout
{
addafter("Order No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Item Journals (83 Item Journal Line) to Item Ledger Entries (32 Item Ledger Entry)
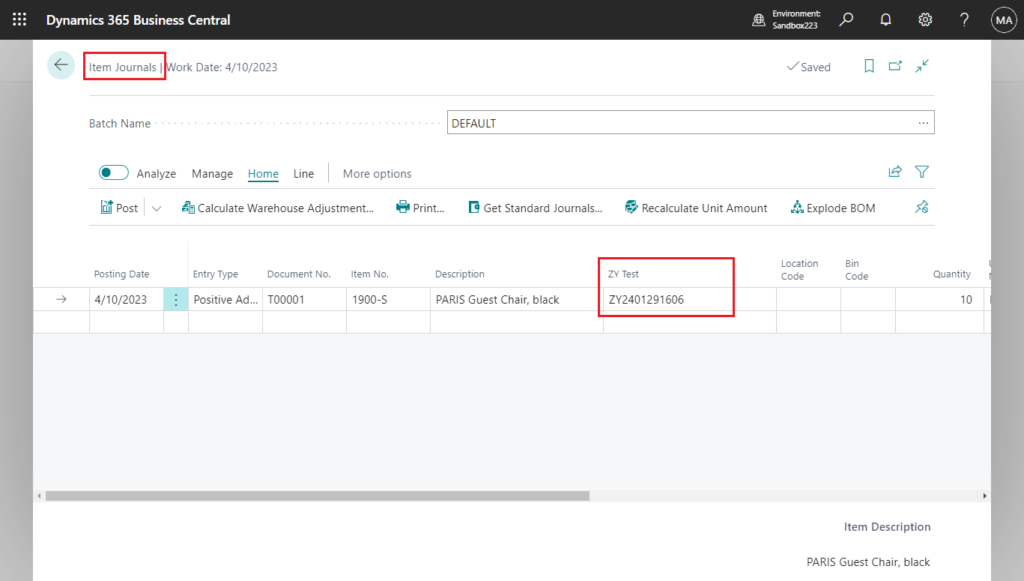

Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Item Jnl.-Post Line", OnAfterInitItemLedgEntry, '', false, false)]
local procedure OnAfterInitItemLedgEntry(var NewItemLedgEntry: Record "Item Ledger Entry"; var ItemJournalLine: Record "Item Journal Line"; var ItemLedgEntryNo: Integer);
begin
NewItemLedgEntry."ZY Test" := ItemJournalLine."ZY Test";
end;
}
tableextension 50122 ItemJournalLineExt extends "Item Journal Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50122 ItemJournalExt extends "Item Journal"
{
layout
{
addafter(Description)
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50113 ItemLedgerEntryExt extends "Item Ledger Entry"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50113 ItemLedgerEntriesExt extends "Item Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Purchase Order Subform (39 Purchase Line) to Item Ledger Entries (32 Item Ledger Entry)
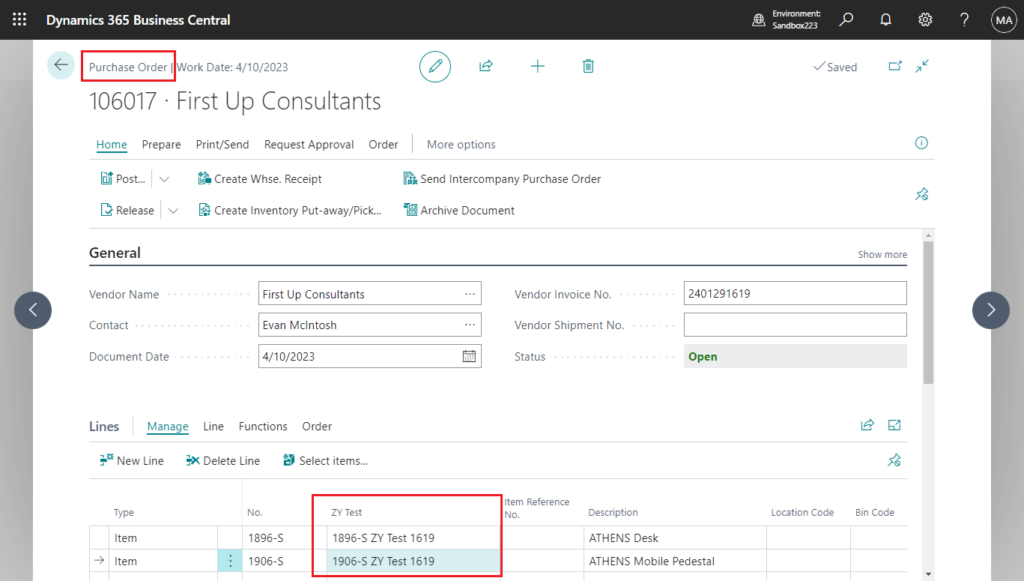
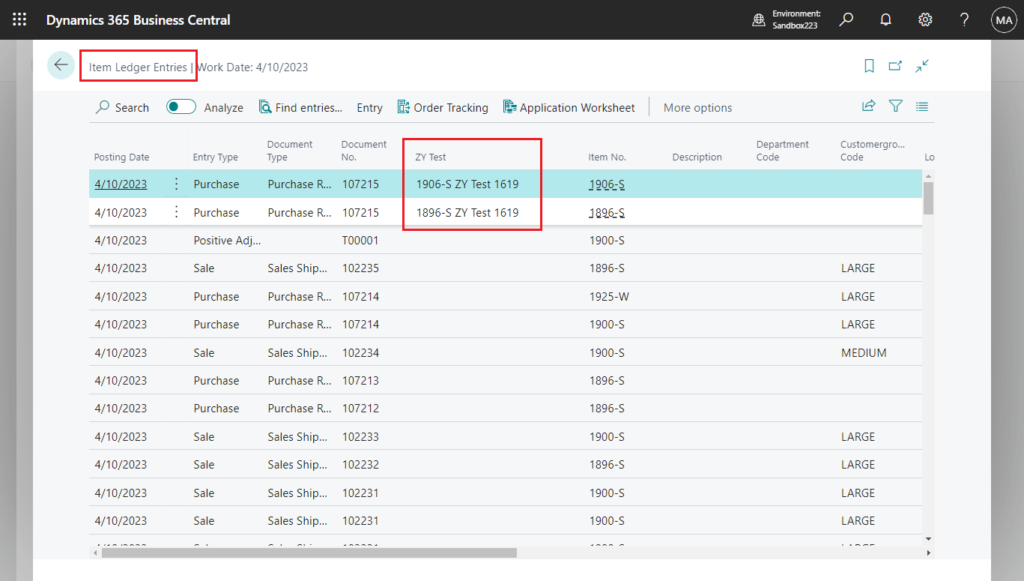
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Purch.-Post", OnPostItemJnlLineOnAfterCopyDocumentFields, '', false, false)]
local procedure OnPostItemJnlLineOnAfterCopyDocumentFields(var ItemJournalLine: Record "Item Journal Line"; PurchaseLine: Record "Purchase Line"; WarehouseReceiptHeader: Record "Warehouse Receipt Header"; WarehouseShipmentHeader: Record "Warehouse Shipment Header"; PurchRcptHeader: Record "Purch. Rcpt. Header");
begin
ItemJournalLine."ZY Test" := PurchaseLine."ZY Test";
end;
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Item Jnl.-Post Line", OnAfterInitItemLedgEntry, '', false, false)]
local procedure OnAfterInitItemLedgEntry(var NewItemLedgEntry: Record "Item Ledger Entry"; var ItemJournalLine: Record "Item Journal Line"; var ItemLedgEntryNo: Integer);
begin
NewItemLedgEntry."ZY Test" := ItemJournalLine."ZY Test";
end;
}
tableextension 50112 PurchaseLineExt extends "Purchase Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50112 PurchaseOrderSubformExt extends "Purchase Order Subform"
{
layout
{
addafter("No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50122 ItemJournalLineExt extends "Item Journal Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
tableextension 50113 ItemLedgerEntryExt extends "Item Ledger Entry"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50113 ItemLedgerEntriesExt extends "Item Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Whse. Receipt Subform (7317 Warehouse Receipt Line) to Item Ledger Entries (32 Item Ledger Entry)
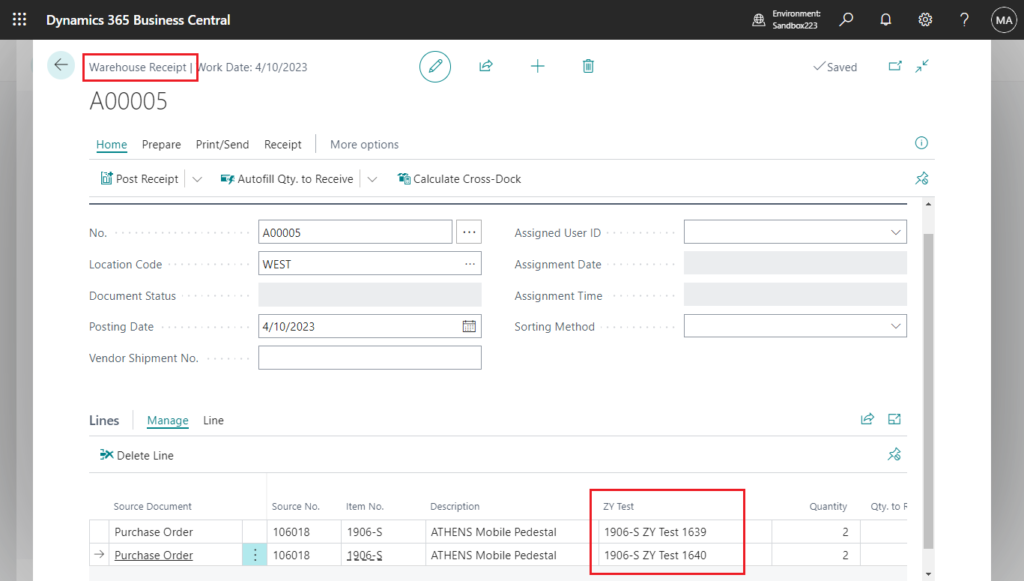
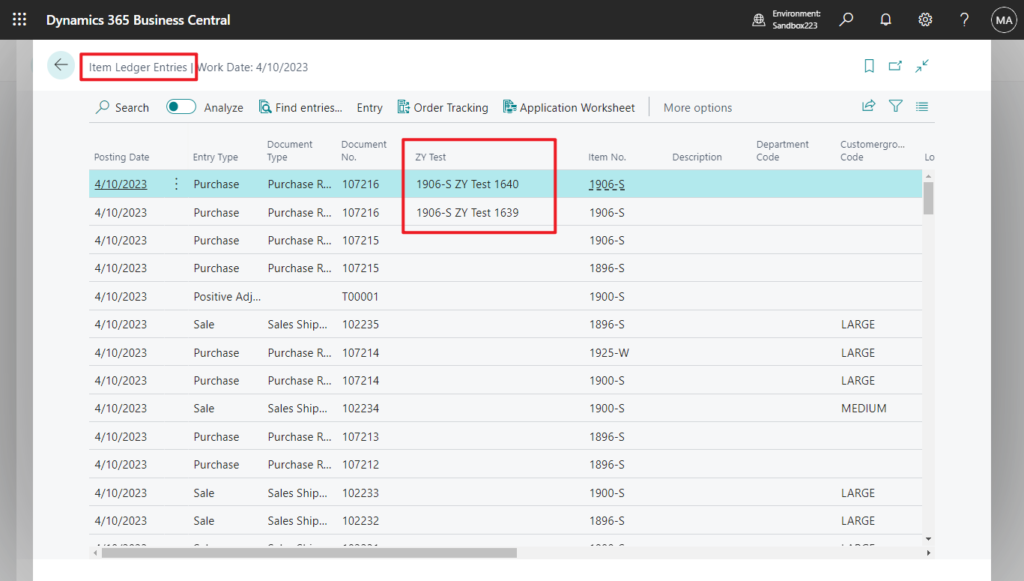
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Whse.-Post Receipt", OnBeforeValidateQtyToReceiveOnPurchaseLine, '', false, false)]
local procedure OnBeforeValidateQtyToReceiveOnPurchaseLine(var PurchaseLine: Record "Purchase Line"; WarehouseReceiptLine: Record "Warehouse Receipt Line"; var IsHandled: Boolean);
begin
PurchaseLine."ZY Test" := WarehouseReceiptLine."ZY Test";
end;
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Purch.-Post", OnPostItemJnlLineOnAfterCopyDocumentFields, '', false, false)]
local procedure OnPostItemJnlLineOnAfterCopyDocumentFields(var ItemJournalLine: Record "Item Journal Line"; PurchaseLine: Record "Purchase Line"; WarehouseReceiptHeader: Record "Warehouse Receipt Header"; WarehouseShipmentHeader: Record "Warehouse Shipment Header"; PurchRcptHeader: Record "Purch. Rcpt. Header");
begin
ItemJournalLine."ZY Test" := PurchaseLine."ZY Test";
end;
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Item Jnl.-Post Line", OnAfterInitItemLedgEntry, '', false, false)]
local procedure OnAfterInitItemLedgEntry(var NewItemLedgEntry: Record "Item Ledger Entry"; var ItemJournalLine: Record "Item Journal Line"; var ItemLedgEntryNo: Integer);
begin
NewItemLedgEntry."ZY Test" := ItemJournalLine."ZY Test";
end;
}
tableextension 50115 WarehouseReceiptLineExt extends "Warehouse Receipt Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50115 WhseReceiptSubformExt extends "Whse. Receipt Subform"
{
layout
{
addafter(Description)
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50114 PurchaseLineExt extends "Purchase Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
tableextension 50112 ItemJournalLineExt extends "Item Journal Line"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
tableextension 50113 ItemLedgerEntryExt extends "Item Ledger Entry"
{
fields
{
field(50000; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50113 ItemLedgerEntriesExt extends "Item Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Sales Journal (81 Gen. Journal Line) to Customer Ledger Entries (21 Cust. Ledger Entry)
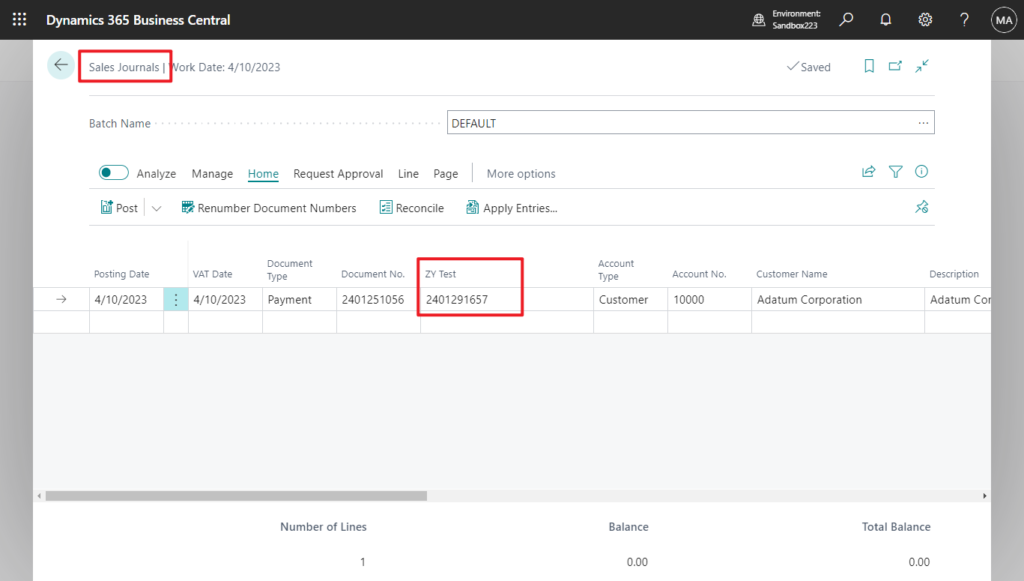
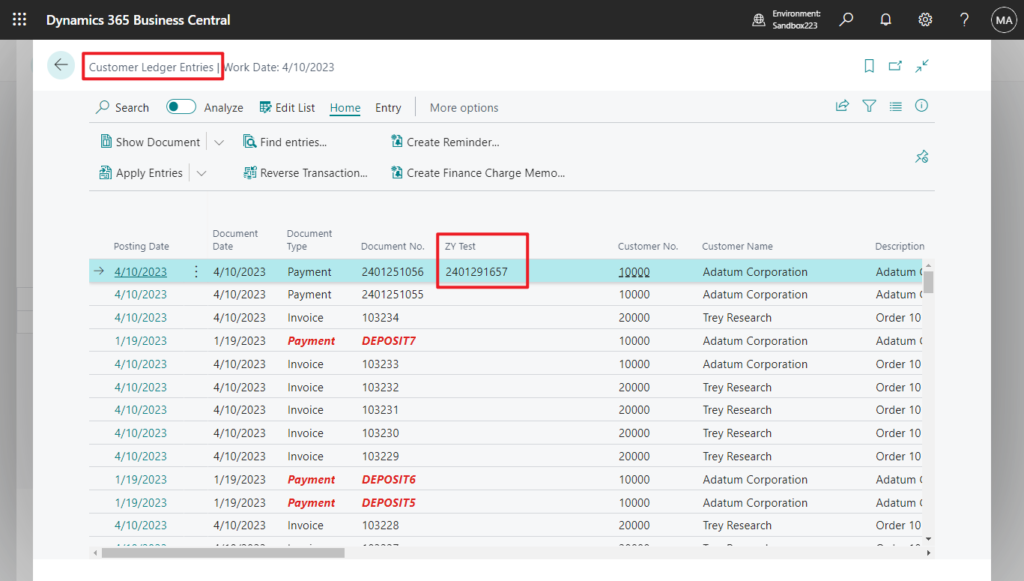
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Gen. Jnl.-Post Line", OnAfterInitCustLedgEntry, '', false, false)]
local procedure OnAfterInitCustLedgEntry(var CustLedgerEntry: Record "Cust. Ledger Entry"; GenJournalLine: Record "Gen. Journal Line"; var GLRegister: Record "G/L Register");
begin
CustLedgerEntry."ZY Test" := GenJournalLine."ZY Test";
end;
}
tableextension 50119 GenJournalLineExt extends "Gen. Journal Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 SalesJournalExt extends "Sales Journal"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50121 CustLedgerEntryExt extends "Cust. Ledger Entry"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 CustomerLedgerEntriesExt extends "Customer Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Purchase Journal (81 Gen. Journal Line) to Vendor Ledger Entries (25 Vendor Ledger Entry)
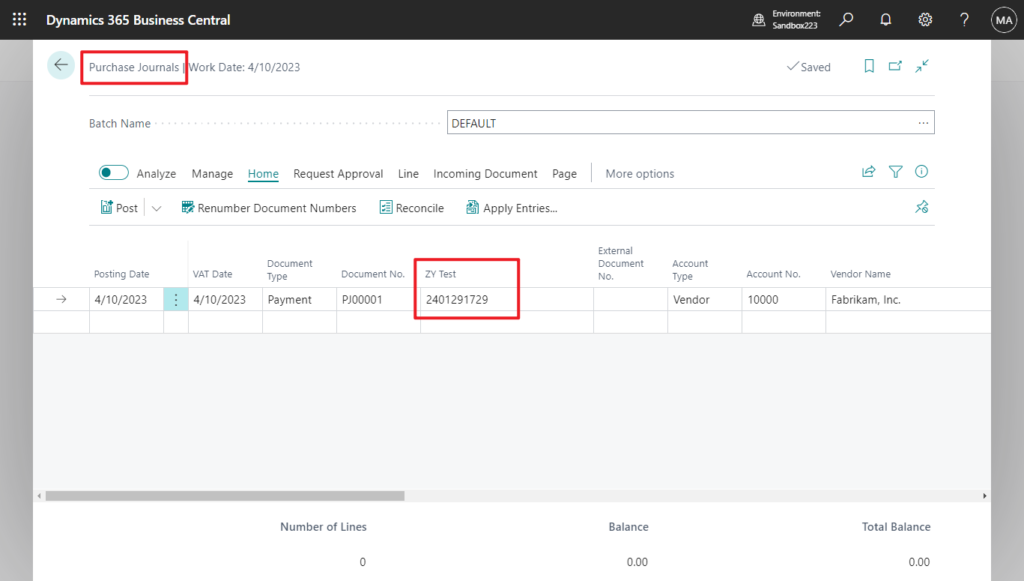
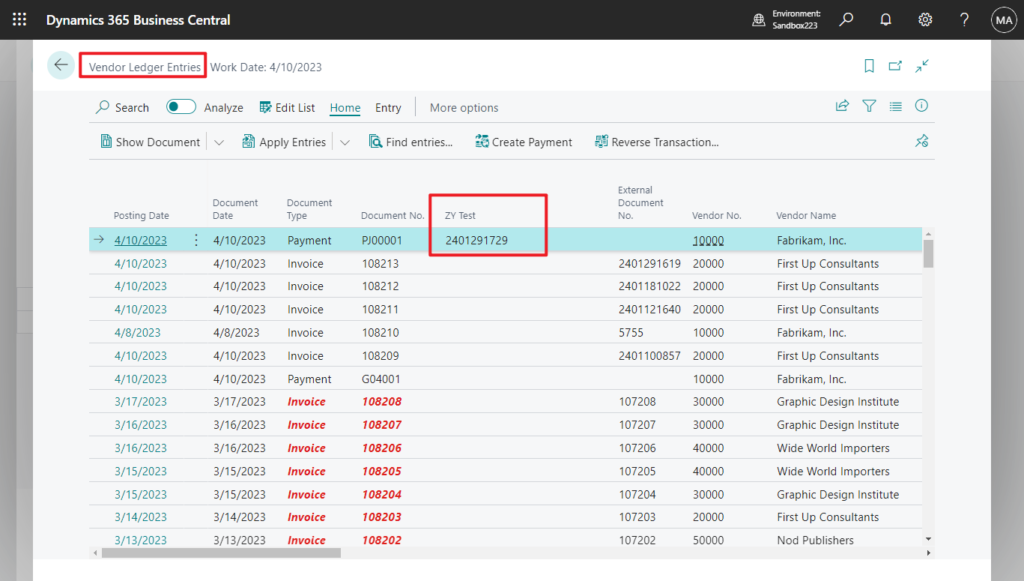
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Table, Database::"Vendor Ledger Entry", OnAfterCopyVendLedgerEntryFromGenJnlLine, '', false, false)]
local procedure OnAfterCopyVendLedgerEntryFromGenJnlLine(var VendorLedgerEntry: Record "Vendor Ledger Entry"; GenJournalLine: Record "Gen. Journal Line");
begin
VendorLedgerEntry."ZY Test" := GenJournalLine."ZY Test";
end;
}
tableextension 50119 GenJournalLineExt extends "Gen. Journal Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 PurchaseJournalExt extends "Purchase Journal"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50121 VendorLedgerEntryExt extends "Vendor Ledger Entry"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 VendorLedgerEntriesExt extends "Vendor Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Purchase Journal (81 Gen. Journal Line) to General Ledger Entries (17 G/L Entry)
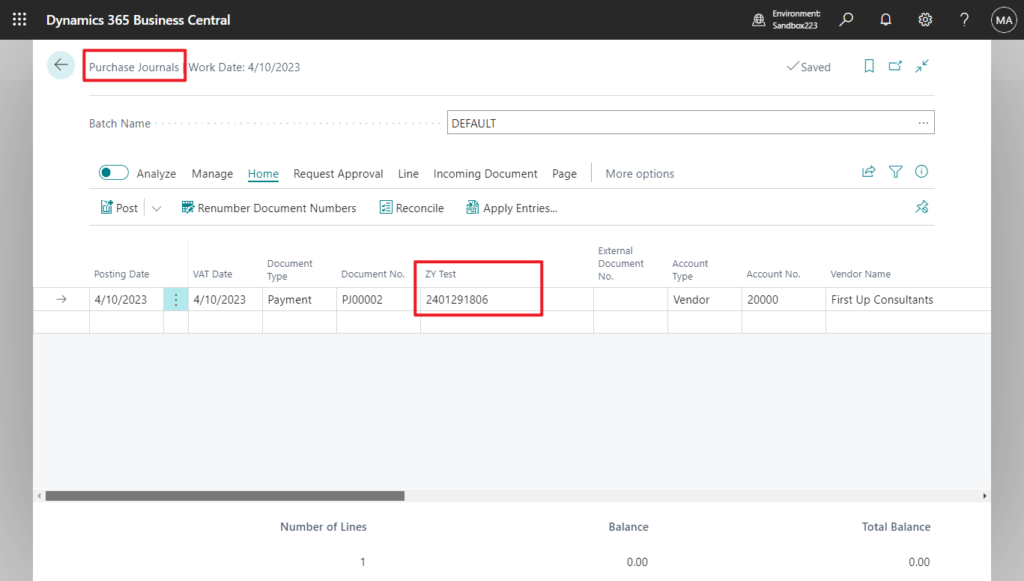
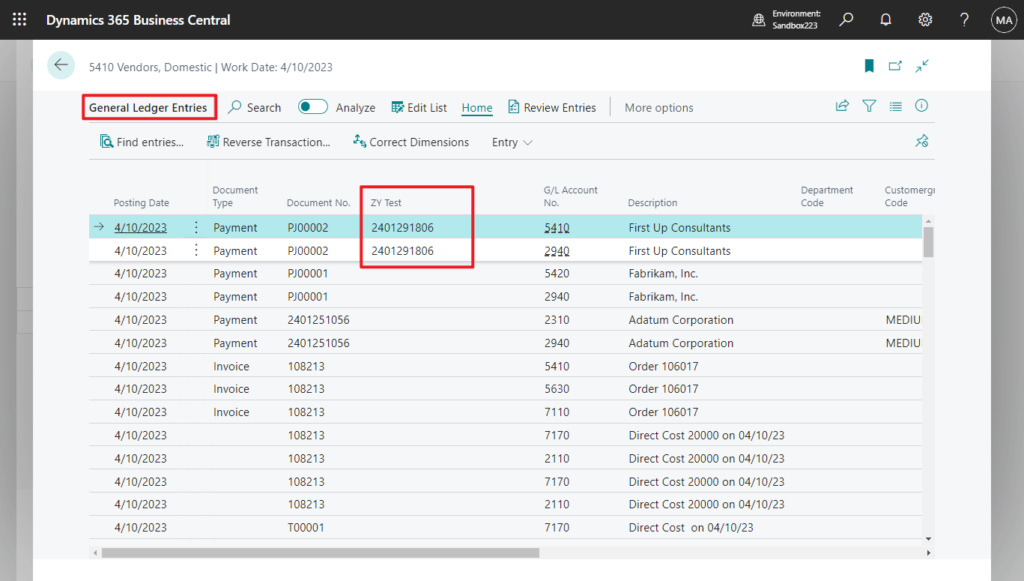
Source code: Github
PS: OnAfterGLEntryFromGenJnlLine -> OnAfterCopyGLEntryFromGenJnlLine
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Table, Database::"G/L Entry", OnAfterCopyGLEntryFromGenJnlLine, '', false, false)]
local procedure OnAfterCopyGLEntryFromGenJnlLine(var GLEntry: Record "G/L Entry"; var GenJournalLine: Record "Gen. Journal Line");
begin
GLEntry."ZY Test" := GenJournalLine."ZY Test";
end;
}
tableextension 50119 GenJournalLineExt extends "Gen. Journal Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 PurchaseJournalExt extends "Purchase Journal"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50121 GLEntryExt extends "G/L Entry"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 GeneralLedgerEntriesExt extends "General Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Sales Order (36 Sales Header) to General Ledger Entries (17 G/L Entry)
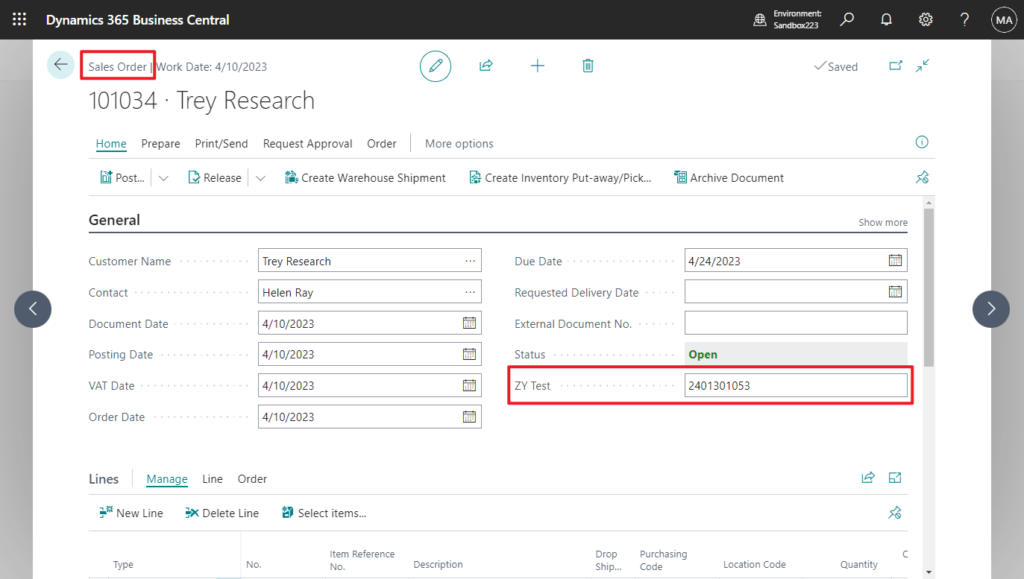
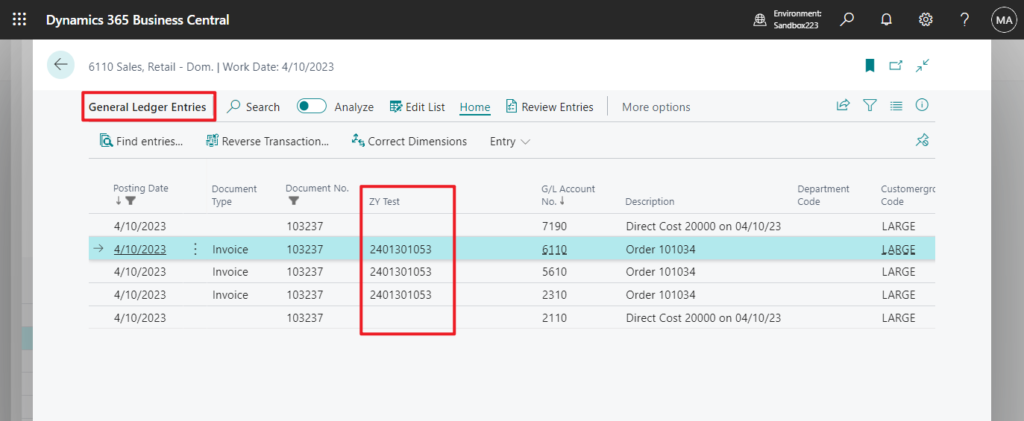
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Table, Database::"Gen. Journal Line", OnAfterCopyGenJnlLineFromSalesHeader, '', false, false)]
local procedure OnAfterCopyGenJnlLineFromSalesHeader(SalesHeader: Record "Sales Header"; var GenJournalLine: Record "Gen. Journal Line");
begin
GenJournalLine."ZY Test" := SalesHeader."ZY Test";
end;
[EventSubscriber(ObjectType::Table, Database::"G/L Entry", OnAfterCopyGLEntryFromGenJnlLine, '', false, false)]
local procedure OnAfterCopyGLEntryFromGenJnlLine(var GLEntry: Record "G/L Entry"; var GenJournalLine: Record "Gen. Journal Line");
begin
GLEntry."ZY Test" := GenJournalLine."ZY Test";
end;
}
tableextension 50119 SalesHeaderExt extends "Sales Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 SalesOrderExt extends "Sales Order"
{
layout
{
addafter(Status)
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50120 GenJournalLineExt extends "Gen. Journal Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
tableextension 50121 GLEntryExt extends "G/L Entry"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 GeneralLedgerEntriesExt extends "General Ledger Entries"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Purchase Order (50 Purchase header) to Warehouse Receipt (7316 Warehouse Receipt Header)
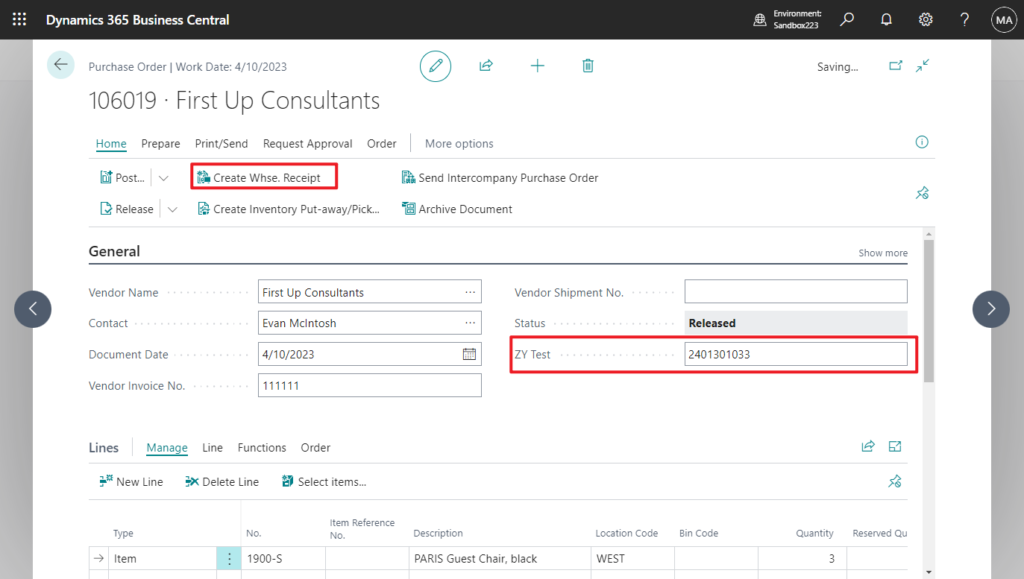
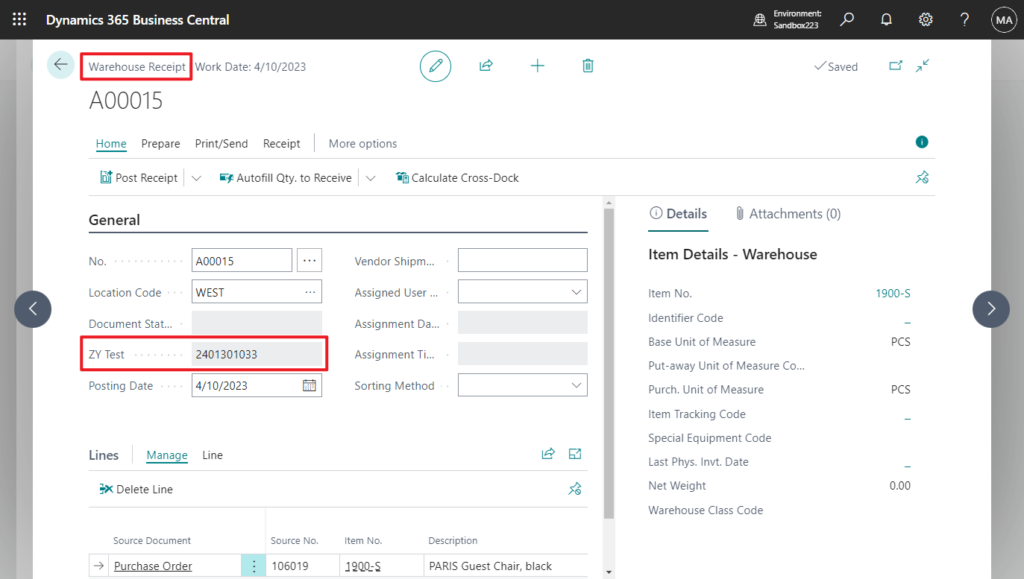
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Get Source Doc. Inbound", OnAfterFindWarehouseRequestForPurchaseOrder, '', false, false)]
local procedure OnAfterFindWarehouseRequestForPurchaseOrder(var WarehouseRequest: Record "Warehouse Request"; PurchaseHeader: Record "Purchase Header");
begin
WarehouseRequest."ZY Test" := PurchaseHeader."ZY Test";
WarehouseRequest.Modify(true);
end;
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Get Source Doc. Inbound", OnAfterCreateWhseReceiptHeaderFromWhseRequest, '', false, false)]
local procedure OnAfterCreateWhseReceiptHeaderFromWhseRequest(var WhseReceiptHeader: Record "Warehouse Receipt Header"; var WarehouseRequest: Record "Warehouse Request"; var GetSourceDocuments: Report "Get Source Documents");
begin
if WhseReceiptHeader."No." <> '' then begin
WhseReceiptHeader."ZY Test" := WarehouseRequest."ZY Test";
WhseReceiptHeader.Modify(true);
end;
end;
}
tableextension 50119 PurchaseHeaderExt extends "Purchase Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 SalesOrderExt extends "Purchase Order"
{
layout
{
addafter(Status)
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50120 WarehouseRequestExt extends "Warehouse Request"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
tableextension 50121 WarehouseReceiptHeaderExt extends "Warehouse Receipt Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 WarehouseReceiptExt extends "Warehouse Receipt"
{
layout
{
addafter("Document Status")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
Editable = false;
}
}
}
}
From Warehouse Shipment (7320 Warehouse Shipment Header) to Posted Sales Shipment (110 Sales Shipment Header)
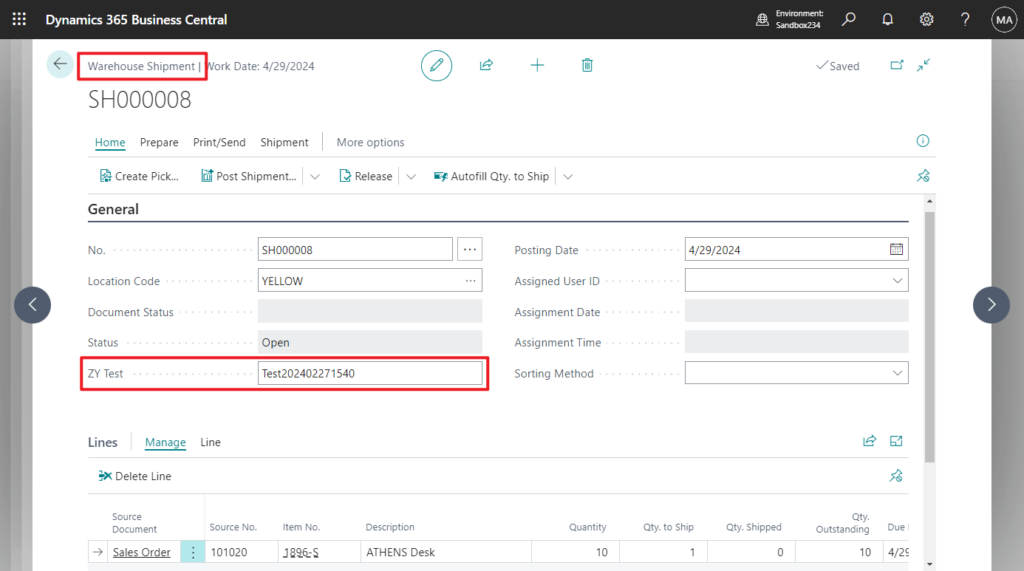
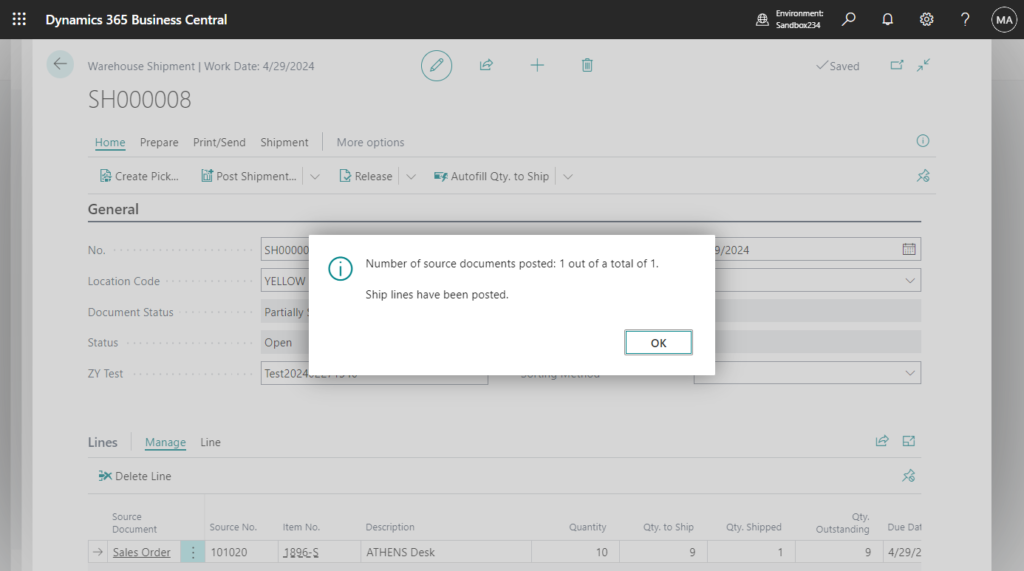
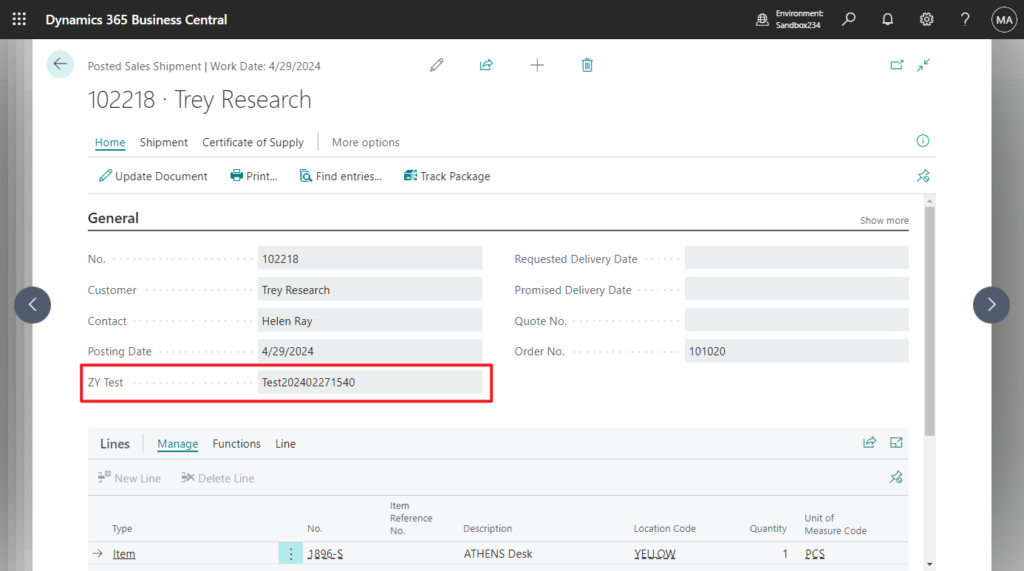
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Codeunit, Codeunit::"Sales-Post", OnBeforeSalesShptHeaderInsert, '', false, false)]
local procedure OnBeforeSalesShptHeaderInsert(var SalesShptHeader: Record "Sales Shipment Header"; SalesHeader: Record "Sales Header"; CommitIsSuppressed: Boolean; var IsHandled: Boolean; var TempWhseRcptHeader: Record "Warehouse Receipt Header" temporary; WhseReceive: Boolean; var TempWhseShptHeader: Record "Warehouse Shipment Header" temporary; WhseShip: Boolean; InvtPickPutaway: Boolean);
begin
SalesShptHeader."ZY Test" := TempWhseShptHeader."ZY Test";
end;
}
tableextension 50119 WarehouseShipmentHeaderExt extends "Warehouse Shipment Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 WarehouseShipmentExt extends "Warehouse Shipment"
{
layout
{
addafter(Status)
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50120 SalesShipmentHeaderExt extends "Sales Shipment Header"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50120 PostedSalesShipmentExt extends "Posted Sales Shipment"
{
layout
{
addafter("Posting Date")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
From Bank Acc. Reconciliation Lines (274 Bank Acc. Reconciliation Line) to General Journal (81 Gen. Journal Line)
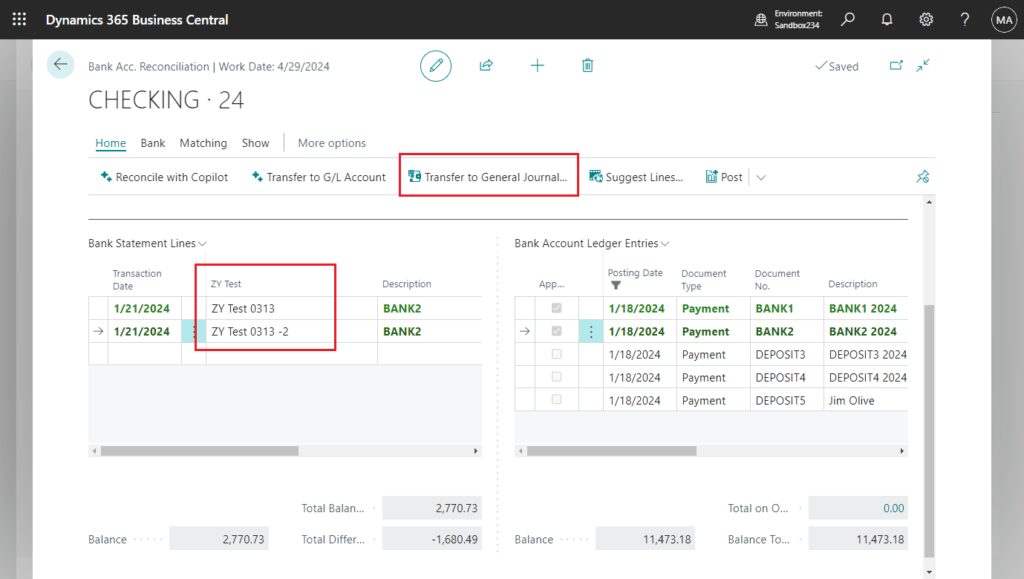
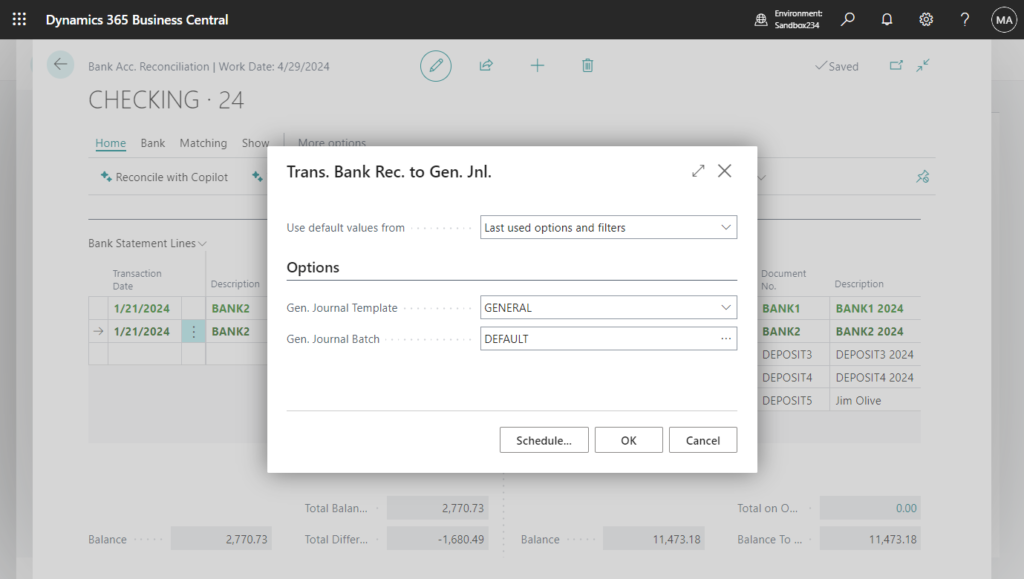
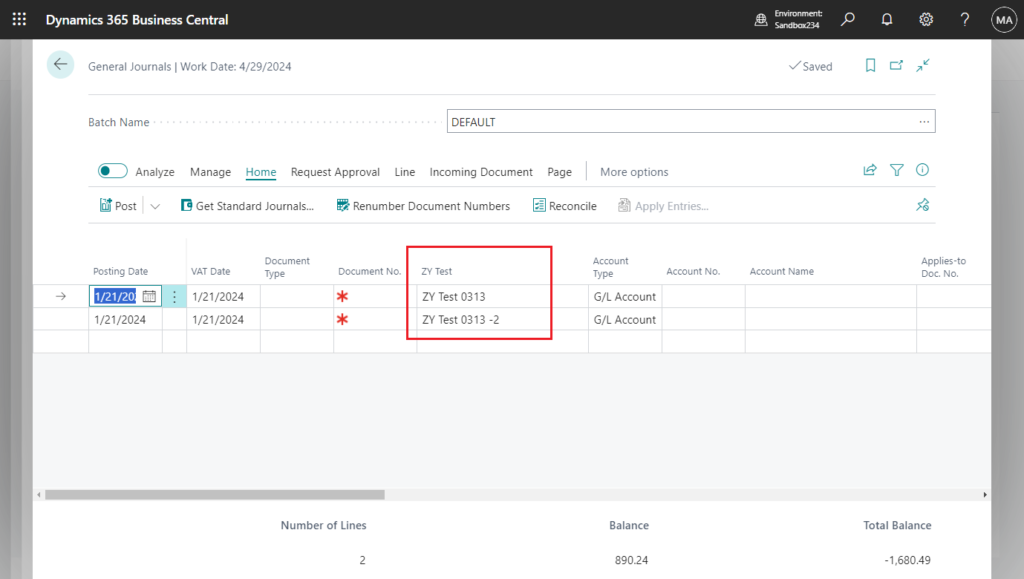
Source code: Github
codeunit 50122 EventHandler
{
[EventSubscriber(ObjectType::Report, Report::"Trans. Bank Rec. to Gen. Jnl.", OnBeforeGenJnlLineInsert, '', false, false)]
local procedure "Trans. Bank Rec. to Gen. Jnl._OnBeforeGenJnlLineInsert"(var GenJournalLine: Record "Gen. Journal Line"; BankAccReconciliationLine: Record "Bank Acc. Reconciliation Line")
begin
GenJournalLine."ZY Test" := BankAccReconciliationLine."ZY Test";
end;
}
tableextension 50119 BankAccReconciliationLineExt extends "Bank Acc. Reconciliation Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50119 BankAccReconciliationLinesExt extends "Bank Acc. Reconciliation Lines"
{
layout
{
addafter("Transaction Date")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
tableextension 50120 GenJournalLineExt extends "Gen. Journal Line"
{
fields
{
field(50100; "ZY Test"; Text[100])
{
Caption = 'ZY Test';
DataClassification = CustomerContent;
}
}
}
pageextension 50121 GeneralJournalExt extends "General Journal"
{
layout
{
addafter("Document No.")
{
field("ZY Test"; Rec."ZY Test")
{
ApplicationArea = All;
}
}
}
}
PS:
1. How to create a Lookup, Drop-Down, or Option list (Single and Multi select)
2. FlowFields (Sum, Average, Exist, Count, Min, Max, Lookup)
END
Hope this will help.
Thanks for your reading.
ZHU
コメント